MICROSERVICES WITH SPRING BOOT TRAINING
- Description
- Reviews
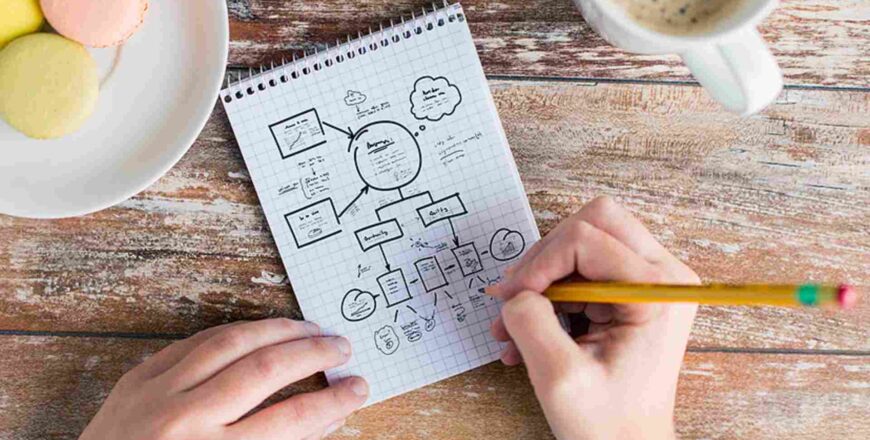
COURSE OVERVIEW
This course covers the essentials of building, deploying, and managing microservices using Spring Boot. Designed for Java developers and software engineers, this hands-on course guides participants through creating scalable, resilient microservices and implementing best practices in communication, security, and monitoring. By the end of this course, attendees will have the skills needed to develop production-ready microservices in Spring Boot, understand service-oriented architecture, and apply key patterns to ensure seamless integration and scalability.
OBJECTIVES
• Microservices Architecture: Understand the principles and benefits of microservices and the differences from monolithic architectures.
• Developing Microservices with Spring Boot: Learn how to create and configure microservices using Spring Boot and Spring Cloud.
• Inter-Service Communication: Master communication between services with REST APIs and messaging with Spring Cloud.
• Microservices Security and Resilience: Implement security and resilience patterns for microservices, including authentication, authorization, and fault tolerance.
• Monitoring, Logging, and Deployment: Learn monitoring, centralized logging, and deployment strategies in a microservices ecosystem.
TARGET AUDIENCE
• Java Developers and Software Engineers: Developers with a background in Java who want to expand into microservices development.
• System Architects: Professionals designing service-oriented or microservices-based systems.
• DevOps Engineers: Engineers responsible for deploying, scaling, and managing microservices in production.
PREREQUISITES
• Familiarity with Java programming and Spring Boot fundamentals is recommended.
• Basic understanding of REST APIs and web services.
TRAINING INCLUSIONS
• Training materials and code samples
• Hands-on labs and exercises
• Certificate of training completion
• 30 Days Post-training Support via LMS
COURSE OUTLINE
Module 1: INTRODUCTION TO MICROSERVICES AND SPRING BOOT
• Understanding Microservices Architecture
- Key Concepts and Principles of Microservices
- Comparing Monolithic and Microservices Architectures
• Introduction to Spring Boot - Overview of Spring Boot Features for Microservices
- Setting Up a Spring Boot Project
Module 2: CREATING MICROSERVICES IN SPRING BOOT
• Service Design and Implementation
- Building Individual Microservices with Spring Boot
- Creating RESTful Endpoints for Service Communication
• Microservices Configuration - Using Spring Boot Profiles for Environment-Specific Configurations
- Externalized Configuration with Spring Cloud Config
Module 3: INTER-SERVICE COMMUNICATION
• REST Communication Between Microservices
- Building REST Clients with Spring RestTemplate and WebClient
• Asynchronous Messaging with Spring Cloud - Implementing Asynchronous Communication with Message Queues (RabbitMQ, Kafka)
- Using Spring Cloud Streams for Event-Driven Communication
• Service Discovery with Eureka - Setting Up Eureka for Service Registration and Discovery
- Using Ribbon for Client-Side Load Balancing
Module 4: MICROSERVICES SECURITY
• Authentication and Authorization
- Implementing Authentication with OAuth2 and JWT
- Role-Based Access Control in Microservices
• API Gateway and Security - Using Spring Cloud Gateway for Secure API Access
- Securing Service-to-Service Communication
Module 5: RESILIENCE AND FAULT TOLERANCE
• Resilience Patterns in Microservices
- Implementing Circuit Breakers with Spring Cloud Circuit Breaker
- Configuring Retry and Timeout Policies with Resilience4j
• Distributed Tracing and Logging - Centralized Logging with ELK Stack or Splunk
- Distributed Tracing with Spring Cloud Sleuth and Zipkin
Module 6: MONITORING AND PERFORMANCE MANAGEMENT
• Monitoring with Micrometer and Prometheus
- Configuring Metrics with Micrometer
- Integrating with Prometheus and Grafana for Visualization
• Health Checks and Alerts - Setting Up Health Checks for Services
- Implementing Alerts and Notifications
Module 7: DEPLOYING MICROSERVICES
• Packaging and Deploying Spring Boot Microservices
- Building Docker Images for Spring Boot Services
- Creating Docker Compose Files for Local Orchestration
• Microservices Deployment on Kubernetes - Overview of Kubernetes Essentials for Microservices
- Deploying Microservices in AKS, EKS, or GKE
Module 8: ADVANCED TOPICS AND BEST PRACTICES
• Event Sourcing and CQRS Patterns
- Understanding Event Sourcing and Command Query Responsibility Segregation
- Implementing Basic CQRS with Spring Boot
• Best Practices for Microservices Development - Strategies for Managing Dependencies and Versioning
- CI/CD Pipeline Integration for Continuous Deployment